In programming, a key factor to consider is how safely a language manages memory. Memory safety means that a programming language helps prevent bugs that can mess up how computer memory is used. This is important because memory safety bugs can lead to crashes and even security issues. In this blog, you will know about the memory safe programming language and its and why it matters.
What is Memory Safety?
Memory safety is a feature in some programming languages that helps prevent bugs related to how computer memory is accessed and stored. These bugs can cause programs to crash or be vulnerable to attacks. Two common types of memory safety bugs are "out of bounds read," where a program tries to access data outside its allocated memory, and "use after free," where a program tries to use memory that has already been freed.
Types of Memory Safety Issues:
Buffer Overflows: This occurs when a program writes more data to a buffer than it can hold. This extra data can overwrite adjacent memory, leading to crashes or vulnerabilities.
Null Pointer Dereferencing: This happens when a program tries to access memory through a null pointer, which can lead to crashes or undefined behavior.
Dangling Pointers: These are pointers that reference memory that has already been freed. Using such pointers can lead to unpredictable behavior and crashes.
Memory Leaks: When a program allocates memory but fails to release it, leading to wasted memory resources and potential performance degradation.
Why Memory Safety is Important
Memory safety is crucial because it helps create more reliable and secure software. Bugs related to memory can be hard to find and fix, and they can lead to serious issues like crashes, data corruption, and security vulnerabilities. By using memory-safe languages, developers can reduce the risk of these bugs and create more robust software.
The Impact of Memory Safety Issues:
Security Vulnerabilities: Many security exploits take advantage of memory safety issues. For example, buffer overflows can be used to inject malicious code into a program.
System Crashes: Memory safety bugs can cause programs or entire systems to crash, leading to downtime and loss of productivity.
Data Corruption: Incorrect memory access can corrupt data, leading to incorrect program behavior and potentially severe consequences, especially in critical systems.
Memory Safe Programming Languages
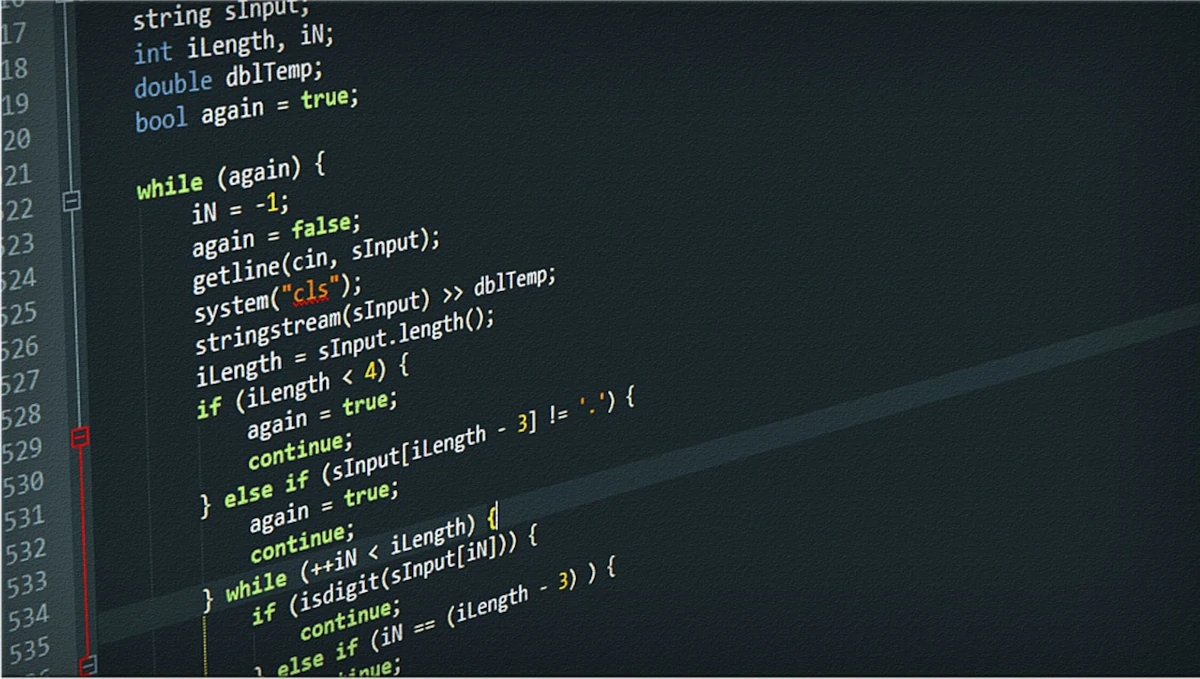
Several programming languages are designed to be memory safe. Here are some of the most popular ones:
1. Rust
Rust is a general-purpose programming language that emphasizes performance, type safety, and concurrency. It enforces memory safety by using a "borrow checker" that ensures all references point to valid memory. Rust is popular for systems programming and has been adopted by companies like Amazon, Google, and Microsoft.
Why Rust is Memory Safe:
Ownership System: Rust's ownership system ensures that each piece of data has a single owner, preventing data races and ensuring memory is properly managed.
Borrow Checker: This feature enforces rules about how data can be borrowed and ensures that no references outlive the data they point to.
No Null Pointers: Rust avoids null pointers, reducing the risk of null pointer dereferencing issues.
2. Python
Python is a high-level, interpreted language known for its simplicity and readability. While Python itself is not memory safe, it encourages good practices that help prevent memory-related bugs. Python's garbage collector helps manage memory automatically, reducing the risk of memory leaks.
Why Python is Memory Safe:
Garbage Collection: Python's automatic garbage collection helps manage memory by reclaiming unused memory, reducing the risk of memory leaks.
High-Level Abstractions: Python provides high-level abstractions that reduce the need for manual memory management, making it easier to write memory-safe code.
3. Java
Java is a widely-used, object-oriented programming language that runs on a virtual machine. Java includes built-in memory management features like garbage collection, which helps prevent memory leaks and other memory-related issues.
Why Java is Memory Safe:
Automatic Memory Management: Java's garbage collector automatically reclaims memory that is no longer needed, reducing the risk of memory leaks.
No Pointer Arithmetic: Java does not allow direct pointer manipulation, reducing the risk of memory safety issues.
Exception Handling: Java's robust exception handling mechanisms help catch and manage errors, reducing the risk of crashes due to memory issues.
4. Go
Go, also known as Golang, is a statically typed, compiled language designed for simplicity and efficiency. Go includes built-in memory management features and encourages safe programming practices.
Why Go is Memory Safe:
Garbage Collection: Go's garbage collector helps manage memory automatically, reducing the risk of memory leaks.
Type Safety: Go's strong typing system helps catch memory-related bugs at compile time.
No Pointer Arithmetic: Like Java, Go does not allow direct pointer manipulation, reducing the risk of memory safety issues.
5. Swift
Swift is a programming language developed by Apple for iOS, macOS, watchOS, and tvOS. Swift includes features like automatic reference counting (ARC) that help manage memory safely and efficiently.
Why Swift is Memory Safe:
Automatic Reference Counting: Swift's ARC automatically manages memory by keeping track of references to objects and deallocating them when they are no longer needed.
Type Safety: Swift's strong type system helps catch memory-related bugs at compile time.
Optional Types: Swift's use of optional types helps prevent null pointer dereferencing issues.
Memory-Unsafe Programming Languages
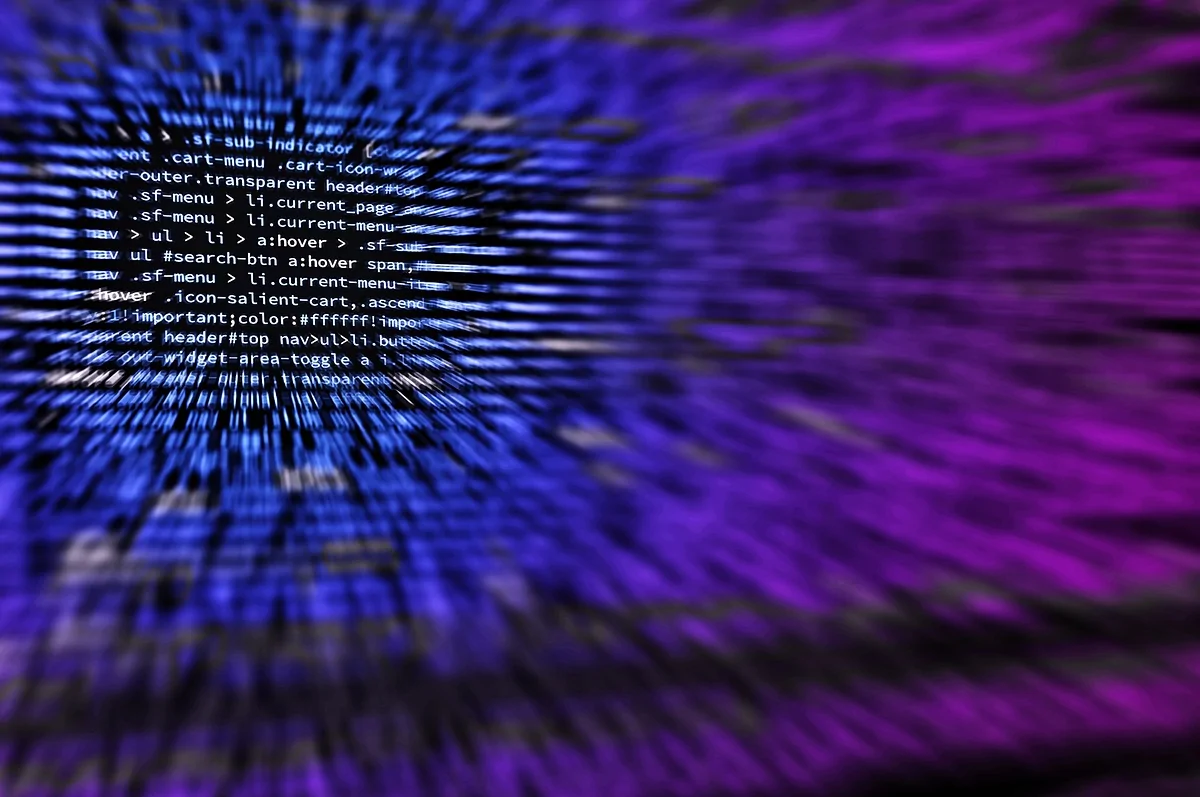
Some programming languages are known for being memory unsafe, meaning they don't have built-in features to prevent memory-related bugs. These languages require developers to manage memory manually, which can lead to errors if not done correctly.
1. C
C is a low-level programming language that gives developers direct control over memory management. While this can be powerful, it also means that developers are responsible for handling memory correctly, which can lead to bugs if not done properly.
Memory Safety Challenges in C:
Manual Memory Management: Developers must manually allocate and free memory, increasing the risk of memory leaks and dangling pointers.
Pointer Arithmetic: C allows direct manipulation of pointers, which can lead to out-of-bounds access and memory corruption.
2. C++
C++ is an extension of C that adds object-oriented features. Like C, C++ requires manual memory management, which can lead to memory-related bugs if not handled carefully.
Memory Safety Challenges in C++:
Manual Memory Management: Like C, C++ requires developers to manage memory manually, increasing the risk of memory leaks and dangling pointers.
Undefined Behavior: C++ allows for low-level memory operations, which can lead to unpredictable behavior, crashes, or security vulnerabilities if not handled correctly.
Use-After-Free: Occurs when a program tries to access memory that has already been freed, leading to potential crashes or security breaches.
Buffer Overflows: Writing more data to a buffer than it can hold can corrupt memory, resulting in crashes or security vulnerabilities.
Dangling Pointers: Arise when an object is deleted, but there are still references to it, leading to unpredictable behavior if the memory is accessed or modified.
Conclusion
Not all memory safe programming language are created equal. Rust stands out as a top choice for system programming due to its strict guarantees, while Go, Java, and Python offer safer alternatives for higher-level applications. However, transitioning to these languages takes time, effort, and resources, especially in industries with significant legacy codebases.
As more organizations recognize the importance of secure software, the shift toward memory-safe languages is gaining momentum. While it may not solve every security problem overnight, adopting these languages is a critical step toward building safer and more reliable software for the future.